Flask Templates
Templates make it easy to write valid HTML. Flask supports my favourite template engine, Jinja2.
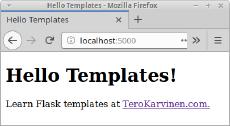
Prerequisites: Hello Flask - Write a Python Web App and Linux command line and administration.
Always start from Hello world. Once that's working, we can add HTML templates.
Directory Structure
Directory structure is pretty simple. HTML templates to to templates/ folder.
$ tree -F
.
├── templates/
│ └── base.html
└── templates-flask.py
Templates-flask.py
Our app has just two new lines compared to "hello world".
#!/usr/bin/python3
"Return HTML templated page"
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def templated():
return render_template("base.html", greeting="Hello Templates!")
app.run(debug=True)
Let's have a look at those new lines
from flask import Flask, render_template
We must import what we use. Render template is imported to main namespace, so we can call it just "render_template()" instead of "flask.render_template".
return render_template("base.html", greeting="Hello Templates!")
In "hello world", we just returned a string 'return "Hello"'. Writing a whole HTML5 page like that would be tedious. Here, we have the HTML template in a separate file.
We declare a new variable called "greeting" for use in the template.
templates/base.html
By default, templates go to templates/ folder.
/2020/flask-templates/02-templates/templates/base.html
<!doctype html>
<html>
<head>
<title>Hello Templates</title>
<meta charset="utf-8">
</head>
<body>
<h1>{{ greeting }}</h1>
<p>
Learn Flask templates at
<a href="http://TeroKarvinen.com">TeroKarvinen.com.</a>
</p>
</body>
</html>
It's just plain HTML, except for the variables.
<h1>{{ greeting }}</h1>
Variables are marked with curly brackets, also known as handlebars, mustache, curly braces (fi: aaltosulkeet). This {{ greeting }} is replaced with the value of the greeting, in this case "Hello Variables!".
Let's Run
$ python3 flask-templates.py
Browse to http://localhost:5000.
That's it. You're now using templates.
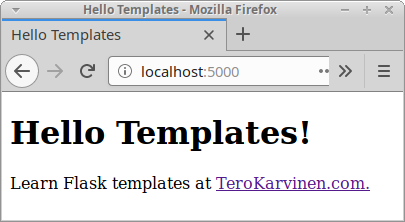
What Next? More Template Tricks
Flask uses my favourite template engine, Jinja2. It has support for a couple of more useful things, which you can learn from Jinja2 documenation.
- Template inheritage. Most of my templates are short, as they inherit the HTML5 boilerplate from base.html. {% extends "base.html" %} {% block content %} Hello {% endblock %}
- Control structures: {% for book in books %} {{ book }} {{ endfor }}
- Filters: {{ message.body|truncate(160) }}
Adminstrivia
This page has been updated.