onAction() Functions - Reacting to Micro Editor Events
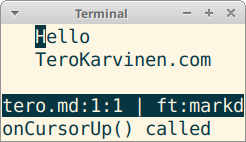
Micro editor plugins can react to user events.
All events that can be bound to keyboard shortcuts, can be used as events in plugins.
function onCursorUp(bp)
-- reacts to CursorUp
end
This post has a table of Micro's every action, onAction function and default keybinding - with explations.
Micro is a TUI text editor with common shortcuts (Ctrl-C, Ctrl-V, Ctrl-S, Ctrl-Q...), advanced features and plugin support. If this is your first Micro plugin, you might want to check out Micro Editor Plugin - a Hello World Tutorial first.
A Sample onAction() event plugin
This sample plugin reacts to cursorUp and cursorDown events. When user moves by pressing up and down arrows, a message is printed on the info bar at the bottom of the screen.
-- main.lua
-- React to user actions with onAction(bp) functions
-- Copyright 2022 Tero Karvinen http://TeroKarvinen.com
local micro = import("micro")
function init()
micro.InfoBar():Message("onAction() demo initialized! See you at TeroKarvinen.com/micro")
end
function onCursorUp(bp)
micro.InfoBar():Message("onCursorUp() called")
end
function onCursorDown(bp)
micro.InfoBar():Message("onCursorDown() called")
end
The key feature is onAction() functions, where the action is any bindable event. For example
function onCursorUp(bp)
-- ...
end
onCursorUp(bp) works without importing anything. The name comes directly from keyboard event.
In official Micro documentation, onAction() functions are described in plugins.md (Ctrl-E help plugins) under "Lua callbacks". All bindable keyboard events are listed in keybindings.md (Ctrl-E help keybindings).
For example, you can react to bindable action "CursorUp" by defining "function onCursorUp(bp)" in your own plugin. The event will work in micro anyway, your function does not prevent it from propagating.
Create the plugin
Create the plugin directory
$ mkdir -p $HOME/.config/micro/plug/onaction/
$ cd $HOME/.config/micro/plug/onaction/
Write the above main.lua into plugin directory onaction/
$ micro main.lua
And put a suitable repo.json in place
[{
"Name": "onaction",
"Description": "React to user actions with onAction(bp) functions",
"Website": "https://terokarvinen.com/tags/micro-editor/",
"Tags": ["event", "onaction", "tutorial", "example"],
"Versions": [
{
"Version": "0.0.1",
"Require": {
"micro": ">=2.0.0"
}
}
]
}]
Try it out
Open micro, write some text, press up and down arrows on your keyboard.
A text at the bottom info bar confirms that you're catching the correct events.
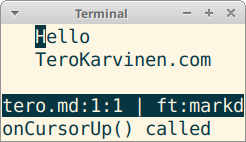
Well done, you can now capture events.
All Micro onAction(bp) functions
Actions are defined in internal/action/actions.go in Micro source code. Each function has a short description as a GoDoc style comment.
One action, suspend, is defined in its own file. Suspend is what would happen to a typical program if user pressed Ctrl-Z. Then it could be resumed with 'fg'. But Micro does not bind suspend to anything.
Micro keybindings can have a context. Source file internal/action/defaults_other.go categorizes the bindings to two categories, bufdefaults and infodefaults. This separation is not noted in the table below.
Initially, I created a list of these functions by taking all bindable actions from keybindings.md (Ctrl-E help keybindings), and added "on" to the start and "(bp") to the end. E.g. "CursorUp" -> "onCursorUp(bp)". But eventually I needed the descriptions and the keybindings, too. I also wanted to be sure that I had all the actions. So I used the latest Micro source code from Github, and wrote a hackish Python+Bash script to collect the data.
The list of actions is in this format: Action description. onAction() function Keybindings.
Rare
Suspend sends micro to the background. This is the same as pressing CtrlZ in most unix programs.. This only works on linux and has no default binding.. This code was adapted from the suspend code in nsf/godit. onSuspend(bp)
None is an action that does nothing. onNone(bp)
Misc
Escape leaves current mode. onEscape(bp) Esc
Deselect deselects on the current cursor. onDeselect(bp) Esc
Scrolling and Cursor movement
ScrollUp is not an action. ScrollUpAction scrolls the view up. onScrollUp(bp) MouseWheelUp
ScrollDown is not an action. ScrollDownAction scrolls the view up. onScrollDown(bp) MouseWheelDown
ScrollAdjust can be used to shift the view so that the last line is at the. bottom if the user has scrolled past the last line. onScrollAdjust(bp)
ScrollUpAction scrolls the view up. onScrollUpAction(bp)
ScrollDownAction scrolls the view up. onScrollDownAction(bp)
Center centers the view on the cursor. onCenter(bp)
MoveCursorUp is not an action. onMoveCursorUp(bp)
MoveCursorDown is not an action. onMoveCursorDown(bp)
CursorUp moves the cursor up. onCursorUp(bp) AltShiftUp, Up
CursorDown moves the cursor down. onCursorDown(bp) AltShiftDown, Down
CursorLeft moves the cursor left. onCursorLeft(bp) Left
CursorRight moves the cursor right. onCursorRight(bp) Right
WordRight moves the cursor one word to the right. onWordRight(bp) Alt-f, AltShiftRight, Ctrl-f, CtrlRight, CtrlShiftRight
WordLeft moves the cursor one word to the left. onWordLeft(bp) Alt-b, Alt-Backspace, Alt-CtrlH, AltShiftLeft, Ctrl-b, Ctrl-d, CtrlLeft, CtrlShiftLeft, Ctrl-w
StartOfText moves the cursor to the start of the text of the line. StartOfTextToggle toggles the cursor between the start of the text of the line. and the start of the line. onStartOfText(bp) Alt-a, AltLeft, AltShiftLeft, CtrlShiftLeft, Home, ShiftHome
StartOfTextToggle toggles the cursor between the start of the text of the line. and the start of the line. onStartOfTextToggle(bp) AltLeft, AltShiftLeft, CtrlShiftLeft, Home, ShiftHome
StartOfLine moves the cursor to the start of the line. onStartOfLine(bp) Ctrl-a
EndOfLine moves the cursor to the end of the line. onEndOfLine(bp) Alt-e, AltRight, AltShiftRight, Ctrl-e, CtrlShiftRight, End, ShiftEnd
ParagraphPrevious moves the cursor to the previous empty line, or beginning of the buffer if there's none. onParagraphPrevious(bp) Alt-{
ParagraphNext moves the cursor to the next empty line, or end of the buffer if there's none. onParagraphNext(bp) Alt-}
CursorStart moves the cursor to the start of the buffer. onCursorStart(bp) AltUp, CtrlHome, CtrlUp
CursorEnd moves the cursor to the end of the buffer. onCursorEnd(bp) AltDown, CtrlDown, CtrlEnd
JumpToMatchingBrace moves the cursor to the matching brace if it is. currently on a brace. onJumpToMatchingBrace(bp)
. onJumpLine(bp)
StartOfText moves the cursor to the start of the text of the line. StartOfTextToggle toggles the cursor between the start of the text of the line. and the start of the line. StartOfLine moves the cursor to the start of the line. Start moves the viewport to the start of the buffer. onStart(bp) Alt-a, AltLeft, AltShiftLeft, AltUp, Ctrl-a, CtrlHome, CtrlShiftLeft, CtrlShiftUp, Ctrl-u, CtrlUp, Home, ShiftHome
EndOfLine moves the cursor to the end of the line. End moves the viewport to the end of the buffer. onEnd(bp) AltDown, Alt-e, AltRight, AltShiftRight, CtrlDown, Ctrl-e, CtrlEnd, CtrlShiftDown, CtrlShiftRight, End, ShiftEnd
PageUp scrolls the view up a page. onPageUp(bp) CtrlPageUp, PageUp
PageDown scrolls the view down a page. onPageDown(bp) CtrlPageDown, PageDown
CursorPageUp places the cursor a page up. onCursorPageUp(bp) PageUp
CursorPageDown places the cursor a page up. onCursorPageDown(bp) PageDown
HalfPageUp scrolls the view up half a page. onHalfPageUp(bp)
HalfPageDown scrolls the view down half a page. onHalfPageDown(bp)
Selection
SelectUp selects up one line. onSelectUp(bp) ShiftUp
SelectDown selects down one line. onSelectDown(bp) ShiftDown
SelectLeft selects the character to the left of the cursor. onSelectLeft(bp) ShiftLeft
SelectRight selects the character to the right of the cursor. onSelectRight(bp) ShiftRight
SelectWordRight selects the word to the right of the cursor. onSelectWordRight(bp) AltShiftRight, CtrlShiftRight
SelectWordLeft selects the word to the left of the cursor. onSelectWordLeft(bp) AltShiftLeft, CtrlShiftLeft
SelectLine selects the entire current line. onSelectLine(bp)
SelectToStartOfText selects to the start of the text on the current line. SelectToStartOfTextToggle toggles the selection between the start of the text. on the current line and the start of the line. onSelectToStartOfText(bp) AltShiftLeft, CtrlShiftLeft, ShiftHome
SelectToStartOfTextToggle toggles the selection between the start of the text. on the current line and the start of the line. onSelectToStartOfTextToggle(bp) AltShiftLeft, CtrlShiftLeft, ShiftHome
SelectToStartOfLine selects to the start of the current line. onSelectToStartOfLine(bp)
SelectToEndOfLine selects to the end of the current line. onSelectToEndOfLine(bp) AltShiftRight, CtrlShiftRight, ShiftEnd
SelectToStartOfText selects to the start of the text on the current line. SelectToStartOfTextToggle toggles the selection between the start of the text. on the current line and the start of the line. SelectToStartOfLine selects to the start of the current line. SelectToStart selects the text from the cursor to the start of the buffer. onSelectToStart(bp) AltShiftLeft, CtrlShiftLeft, CtrlShiftUp, Ctrl-u, ShiftHome
SelectToEndOfLine selects to the end of the current line. SelectToEnd selects the text from the cursor to the end of the buffer. onSelectToEnd(bp) AltShiftRight, CtrlShiftDown, CtrlShiftRight, ShiftEnd
SelectAll selects the entire buffer. onSelectAll(bp) Ctrl-a
SelectPageUp selects up one page. onSelectPageUp(bp)
SelectPageDown selects down one page. onSelectPageDown(bp)
Mouse Events
MousePress is the event that should happen when a normal click happens. This is almost always bound to left click. onMousePress(bp) MouseLeft
Modify text
Retab changes all tabs to spaces or all spaces to tabs depending. on the user's settings. onRetab(bp)
InsertNewline inserts a newline plus possible some whitespace if autoindent is on. onInsertNewline(bp) Enter
Backspace deletes the previous character. onBackspace(bp) Alt-Backspace, Backspace, CtrlH, OldBackspace
DeleteWordRight deletes the word to the right of the cursor. onDeleteWordRight(bp)
DeleteWordLeft deletes the word to the left of the cursor. onDeleteWordLeft(bp) Alt-Backspace, Alt-CtrlH, Ctrl-d, Ctrl-w
DeleteWordRight deletes the word to the right of the cursor. DeleteWordLeft deletes the word to the left of the cursor. Delete deletes the next character. DeleteLine deletes the current line. Delete and paste if the user has a selection. onDelete(bp) Alt-Backspace, Alt-CtrlH, Ctrl-d, Ctrl-w, Delete
IndentSelection indents the current selection. onIndentSelection(bp) Tab
IndentLine moves the current line forward one indentation. onIndentLine(bp)
OutdentLine moves the current line back one indentation. onOutdentLine(bp) Backtab
OutdentSelection takes the current selection and moves it back one indent level. onOutdentSelection(bp) Backtab
InsertTab inserts a tab or spaces. onInsertTab(bp) Tab
DeleteLine deletes the current line. onDeleteLine(bp)
MoveLinesUp moves up the current line or selected lines if any. onMoveLinesUp(bp) AltUp
MoveLinesDown moves down the current line or selected lines if any. onMoveLinesDown(bp) AltDown
Autocomplete
Autocomplete cycles the suggestions and performs autocompletion if there are suggestions. onAutocomplete(bp) Backtab, Tab
CycleAutocompleteBack cycles back in the autocomplete suggestion list. onCycleAutocompleteBack(bp) Backtab
File: New, Save, Quit
SaveAll saves all open buffers. onSaveAll(bp)
SaveCB performs a save and does a callback at the very end (after all prompts have been resolved). onSaveCB(bp)
SaveAll saves all open buffers. SaveCB performs a save and does a callback at the very end (after all prompts have been resolved). Save the buffer to disk. SaveAsCB performs a save as and does a callback at the very end (after all prompts have been resolved). The callback is only called if the save was successful. SaveAs saves the buffer to disk with the given name. onSave(bp) Ctrl-s, F2
SaveAsCB performs a save as and does a callback at the very end (after all prompts have been resolved). The callback is only called if the save was successful. onSaveAsCB(bp)
SaveAsCB performs a save as and does a callback at the very end (after all prompts have been resolved). The callback is only called if the save was successful. SaveAs saves the buffer to disk with the given name. onSaveAs(bp)
. onsaveBufToFile(bp)
OpenFile opens a new file in the buffer. OpenFile opens a new file in the buffer. onOpenFile(bp) Ctrl-o
ForceQuit closes the current tab or view even if there are unsaved changes. (no prompt). onForceQuit(bp)
Quit this will close the current tab or view that is open. QuitAll quits the whole editor; all splits and tabs. onQuit(bp) Ctrl-q, F10, F4
QuitAll quits the whole editor; all splits and tabs. onQuitAll(bp)
Tabs and Splits
AddTab adds a new tab with an empty buffer. onAddTab(bp) Ctrl-t
PreviousTab switches to the previous tab in the tab list. onPreviousTab(bp) Alt-,, CtrlPageUp
NextTab switches to the next tab in the tab list. onNextTab(bp) Alt-., CtrlPageDown
VSplitAction opens an empty vertical split. onVSplitAction(bp)
HSplitAction opens an empty horizontal split. onHSplitAction(bp)
Unsplit closes all splits in the current tab except the active one. onUnsplit(bp)
NextSplit changes the view to the next split. onNextSplit(bp) Ctrl-w
PreviousSplit changes the view to the previous split. onPreviousSplit(bp)
Search and Replace
Find opens a prompt and searches forward for the input. FindLiteral is the same as Find() but does not support regular expressions. FindNext searches forwards for the last used search term. FindPrevious searches backwards for the last used search term. onFind(bp) Alt-F, Ctrl-f, Ctrl-n, Ctrl-p, F3, F7
FindLiteral is the same as Find() but does not support regular expressions. onFindLiteral(bp) Alt-F
Search searches for a given string/regex in the buffer and selects the next. match if a match is found. This function behaves the same way as Find and FindLiteral actions:. it affects the buffer's LastSearch and LastSearchRegex (saved searches). onSearch(bp) Esc
. onfind(bp)
ToggleHighlightSearch toggles highlighting all instances of the last used search term. onToggleHighlightSearch(bp)
UnhighlightSearch unhighlights all instances of the last used search term. onUnhighlightSearch(bp) Esc
FindNext searches forwards for the last used search term. onFindNext(bp) Ctrl-n
FindPrevious searches backwards for the last used search term. onFindPrevious(bp) Ctrl-p
Undo and Redo
Undo undoes the last action. onUndo(bp) Ctrl-z
Redo redoes the last action. onRedo(bp) Ctrl-y
Copy and Paste
Copy the selection to the system clipboard. CopyLine copies the current line to the clipboard. onCopy(bp) Ctrl-c
CopyLine copies the current line to the clipboard. onCopyLine(bp) Ctrl-c
CutLine cuts the current line to the clipboard. onCutLine(bp) Ctrl-k
CutLine cuts the current line to the clipboard. Cut the selection to the system clipboard. onCut(bp) Ctrl-k, Ctrl-x
DuplicateLine duplicates the current line or selection. onDuplicateLine(bp) Ctrl-d
Paste whatever is in the system clipboard into the buffer. Delete and paste if the user has a selection. PastePrimary pastes from the primary clipboard (only use on linux). onPaste(bp) Ctrl-v, MouseMiddle
PastePrimary pastes from the primary clipboard (only use on linux). onPastePrimary(bp) MouseMiddle
. onpaste(bp)
View - Ruler, Gutter, Info bar, Help
ToggleDiffGutter turns the diff gutter off and on. onToggleDiffGutter(bp)
ToggleRuler turns line numbers off and on. onToggleRuler(bp) Ctrl-r
ClearStatus clears the messenger bar. onClearStatus(bp)
ToggleHelp toggles the help screen. onToggleHelp(bp) Ctrl-g
ToggleKeyMenu toggles the keymenu option and resizes all tabs. onToggleKeyMenu(bp) Alt-g
ClearInfo clears the infobar. onClearInfo(bp) Esc
Terminal
ShellMode opens a terminal to run a shell command. onShellMode(bp) Ctrl-b
Command mode
CommandMode lets the user enter a command. onCommandMode(bp) Ctrl-e
ToggleOverwriteMode lets the user toggle the text overwrite mode. onToggleOverwriteMode(bp) Insert
Macros
ToggleMacro toggles recording of a macro. onToggleMacro(bp) Ctrl-u
PlayMacro plays back the most recently recorded macro. onPlayMacro(bp) Ctrl-j
Multicursor
SpawnMultiCursor creates a new multiple cursor at the next occurrence of the current selection or current word. SpawnMultiCursorUp creates additional cursor, at the same X (if possible), one Y less.. SpawnMultiCursorDown creates additional cursor, at the same X (if possible), one Y more.. SpawnMultiCursorSelect adds a cursor at the beginning of each line of a selection. onSpawnMultiCursor(bp) Alt-m, Alt-n, AltShiftDown, AltShiftUp
SpawnMultiCursorUp creates additional cursor, at the same X (if possible), one Y less. onSpawnMultiCursorUp(bp) AltShiftUp
SpawnMultiCursorDown creates additional cursor, at the same X (if possible), one Y more. onSpawnMultiCursorDown(bp) AltShiftDown
SpawnMultiCursorSelect adds a cursor at the beginning of each line of a selection. onSpawnMultiCursorSelect(bp) Alt-m
MouseMultiCursor is a mouse action which puts a new cursor at the mouse position. onMouseMultiCursor(bp) Ctrl-MouseLeft
SkipMultiCursor moves the current multiple cursor to the next available position. onSkipMultiCursor(bp) Alt-x
RemoveMultiCursor removes the latest multiple cursor. onRemoveMultiCursor(bp) Alt-p
RemoveAllMultiCursors removes all cursors except the base cursor. onRemoveAllMultiCursors(bp) Alt-c, Esc