Python Dotted Dictionary
Access your Python dict with a dot
>>> sn.url
'TeroKarvinen.com'
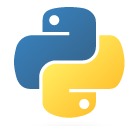
Using just built-in standard libraries
>>> sn = SimpleNamespace(**d)
Converting dict to dotted
$ python3
Create a regular dict
>>> d = {"name": "Tero", "url": "TeroKarvinen.com", "x": 3}
Convert to dot notation
>>> from types import SimpleNamespace
>>> sn = SimpleNamespace(**d)
Access items using dot notation
>>> sn.name
'Tero'
>>> sn.url
'TeroKarvinen.com'
>>> sn.x
3
Dot Notation with f-Strings
Dot notation is very convenient with f-strings. Instead of writing
>>> print(f'{d["name"]} {d["x"]} {d["url"]}"')
Tero 3 TeroKarvinen.com"
You can write
>>> print(f"{sn.name} {sn.x} {sn.url}")
Tero 3 TeroKarvinen.com
Troubleshooting
>>> sn = SimpleNamespace(d)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: no positional arguments expected
You should use double star (double asterisk, **) to unpack dictionary to keyword arguments. SimpleNamespace(**d).